December 25, 2024|7 min reading
How to Use OpenLLM for LLM Development with LangChain: A Step-by-Step Guide
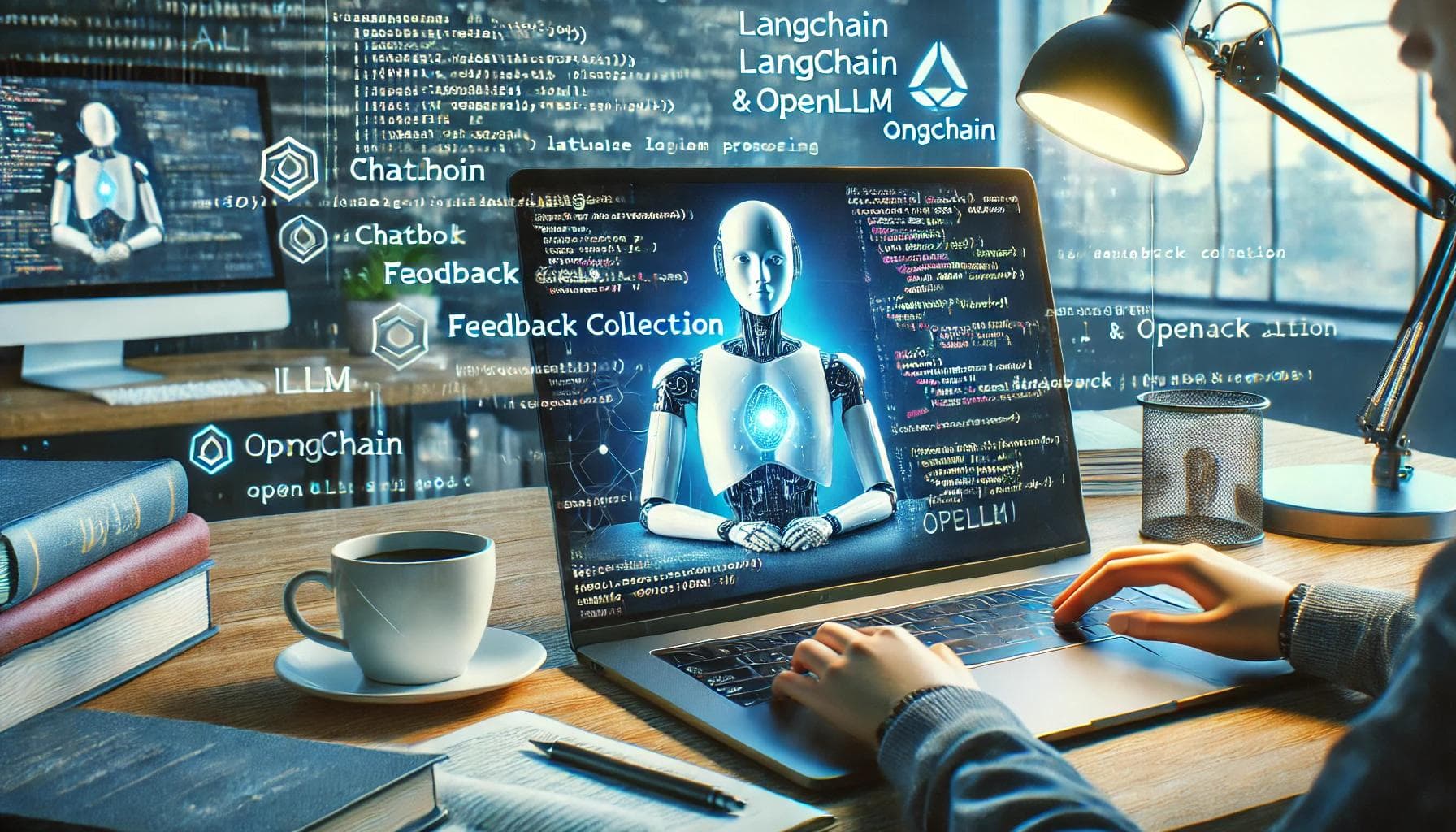
Don't Miss This Free AI!
Unlock hidden features and discover how to revolutionize your experience with AI.
Only for those who want to stay ahead.
Introduction to OpenLLM
In the rapidly advancing field of artificial intelligence, language models are pivotal for driving understanding and interaction across numerous applications. OpenLLM, an open-source framework, offers developers the ability to harness large language models (LLMs) effectively. When paired with LangChain, a library designed to streamline the creation of language-based applications, OpenLLM’s capabilities become even more powerful. This article walks you through how to utilize OpenLLM within the LangChain environment to build your own language applications, from setup to execution.
TL;DR - Quick Overview
- Install Necessary Packages: Use pip to install LangChain and OpenLLM.
- Initialize OpenLLM: Set up OpenLLM with your API key in a Python script.
- Configure LangChain: Integrate OpenLLM as the language model in LangChain.
- Build and Run Your Application: Develop and run a basic language application, such as a chatbot.
What Is OpenLLM and How Does It Help?
OpenLLM serves a variety of roles in the realm of AI and machine learning, including:
- Enhancing text-based applications such as summarization, translation, and question-answering.
- Providing a foundation for developing custom language models tailored to specific needs.
- Enabling efficient deployment and experimentation with large language models for research and development.
How to Use ChatOpenAI in LangChain
LangChain simplifies the development of AI-driven language applications, especially for conversational models. By integrating ChatOpenAI, a component designed to interact with OpenAI’s conversational models, developers can efficiently build and manage AI chat systems. Follow these steps to get started:
Step 1: Setting Up Your Development Environment
Before integrating ChatOpenAI, ensure Python (version 3.7 or newer) is installed. It's best to set up a virtual environment to keep your dependencies organized:
bashCopy code# Create a virtual environment
python -m venv langchain-env
# Activate the virtual environment
# On Windows
langchain-env\Scripts\activate
# On Unix or MacOS
source langchain-env/bin/activate
Step 2: Installing LangChain
With your virtual environment activated, use pip to install LangChain:
bashCopy codepip install langchain
This command installs LangChain and its dependencies.
Step 3: Importing ChatOpenAI
To integrate ChatOpenAI, import it into your script:
pythonCopy codefrom langchain.chat_openai import ChatOpenAI
Step 4: Configuring ChatOpenAI
Initialize ChatOpenAI with your OpenAI API key:
pythonCopy code# Initialize ChatOpenAI with your OpenAI API key
chat_openai = ChatOpenAI(api_key="your_openai_api_key_here")
Replace your_openai_api_key_here with your actual OpenAI API key to authenticate requests.
Step 5: Creating a Conversation
Set up a function that manages the conversation loop. The AI will interact with the user based on their input:
pythonCopy codedef start_conversation():
while True:
user_input = input("You: ")
if user_input.lower() == "quit":
break
response = chat_openai.generate_response(user_input)
print("AI:", response)
Step 6: Running the Chat
To initiate the conversation, simply call the function:
pythonCopy code# Start the conversation
start_conversation()
Running this will start an interactive chat session.
Example: Building a Feedback Collection Bot
Let’s explore how to use LangChain and ChatOpenAI to create a simple feedback collection bot. This bot engages with users, collects feedback, and processes it for sentiment analysis.
Step 1: Setting Up the Bot
Ensure LangChain and ChatOpenAI are correctly installed. Then, initialize the bot with your OpenAI API key:
pythonCopy codefrom langchain.chat_openai import ChatOpenAI
# Initialize ChatOpenAI
chat_openai = ChatOpenAI(api_key="your_openai_api_key_here")
Step 2: Creating the Interaction Logic
Create the bot’s interaction logic to collect and analyze user feedback:
pythonCopy codedef feedback_bot():
print("Hello! How was your experience with our service today?")
while True:
feedback = input("Your feedback: ")
if feedback.lower() == "quit":
break
analyze_feedback(feedback)
Step 3: Analyzing Feedback
Implement a basic keyword-based sentiment analysis to classify feedback:
pythonCopy codedef analyze_feedback(feedback):
positive_keywords = ["great", "excellent", "good", "fantastic", "happy"]
negative_keywords = ["bad", "poor", "terrible", "unhappy", "worst"]
if any(word in feedback.lower() for word in positive_keywords):
print("AI: We're thrilled to hear that! Thank you for your feedback.")
elif any(word in feedback.lower() for word in negative_keywords):
print("AI: We're sorry to hear that. We'll work on improving.")
else:
print("AI: Thank you for your feedback. We're always looking to improve.")
Step 4: Enhancing with Advanced Sentiment Analysis
For more nuanced analysis, you can integrate advanced models to better interpret sentiment and generate personalized responses. LangChain makes it easy to switch to more complex models for enhanced performance.
Troubleshooting: Cannot Run OpenLLM? Check Your Python Version
OpenLLM requires Python 3.7 or higher. To check your Python version, run the following command:
bashCopy codepython --version
If your Python version is outdated, update it to a compatible version.
Conclusion
Integrating OpenLLM with LangChain enables developers to create powerful language applications, from simple chatbots to sophisticated AI-driven tools. By following the steps outlined in this guide, you can enhance your projects with OpenLLM’s capabilities and streamline development with LangChain’s intuitive API.
FAQ
1. What is OpenLLM?
OpenLLM is an open-source framework that helps developers utilize large language models in AI applications.
2. Can I use OpenLLM for non-conversational applications?
Yes, OpenLLM can be used for a wide range of language-based tasks such as summarization, translation, and custom model development.
3. Do I need coding experience to use LangChain?
While some basic programming knowledge is helpful, LangChain is designed to be accessible to developers at all levels, with ample documentation and examples.
4. What if I face compatibility issues with OpenLLM?
Ensure you are using Python 3.7 or a newer version. If you continue to face issues, consider upgrading your Python installation.
Explore more
16 Best SEO Content Writing Tools to Boost Your Rankings
Discover the 16 best SEO content writing tools to optimize your workflow, enhance content quality, and boost your searc...
SEO Writing: Proven Tips for Creating SEO-Optimized Content
Learn expert SEO writing strategies to improve search rankings and boost traffic. Optimize your content with our proven...
How to Build Effective Topic Clusters for Maximum SEO Impact
Learn how to create effective topic clusters to boost your SEO strategy and improve search rankings with this step-by-st...