December 25, 2024|8 min reading
How to Build an AI Coding Assistant with Llama 3
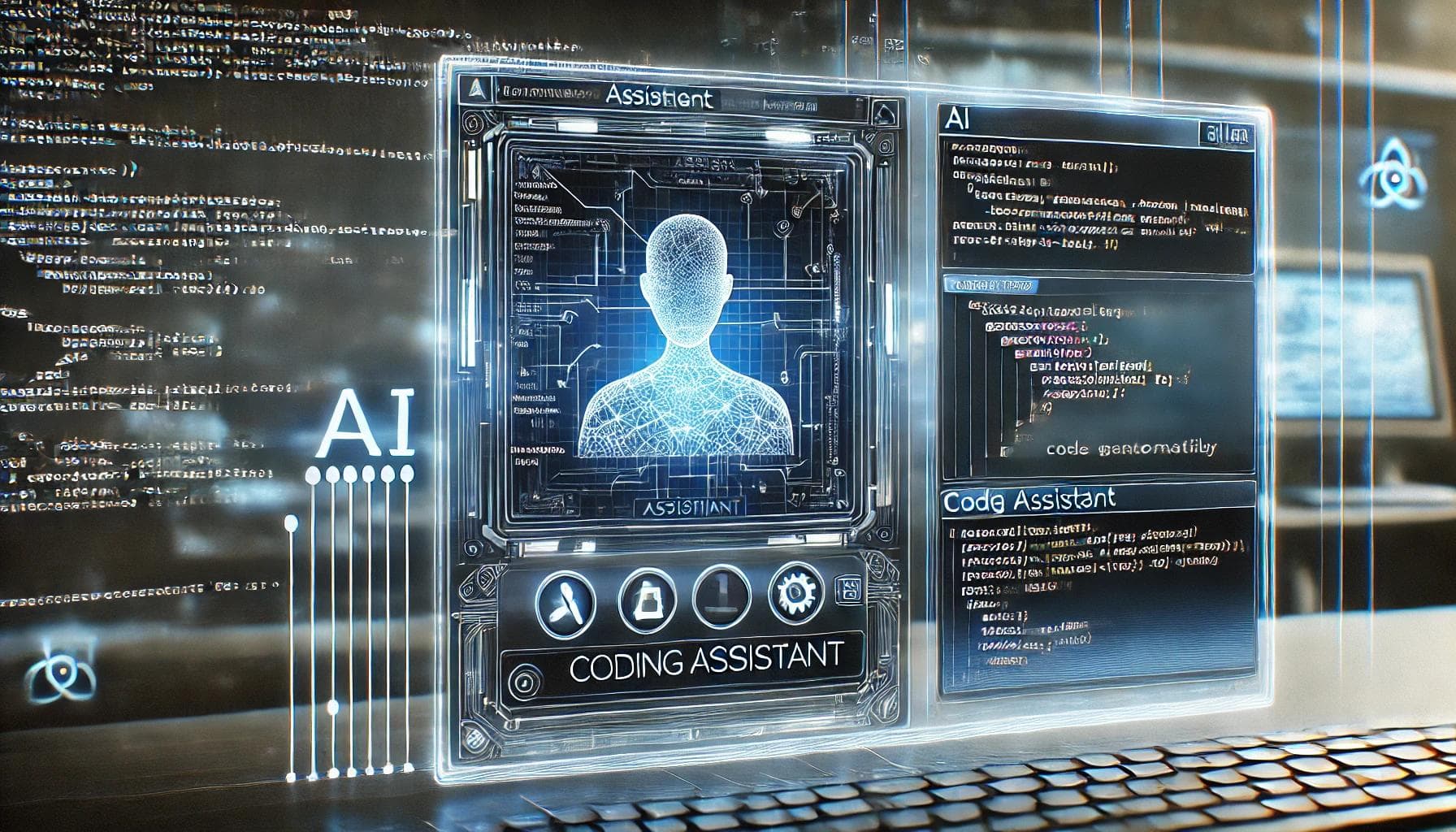
Don't Miss This Free AI!
Unlock hidden features and discover how to revolutionize your experience with AI.
Only for those who want to stay ahead.
Building an AI-powered coding assistant is no longer just a dream. With the release of Llama 3 by Meta, developers now have access to one of the most powerful large language models (LLMs) designed for a variety of tasks—including code generation and logical reasoning. This guide will walk you through creating your own AI coding assistant using Llama 3. Whether you're a beginner or an experienced developer, this comprehensive tutorial provides you with the tools to build a useful AI tool for your coding projects.
TL;DR
- Download and install Llama 3 from Meta's GitHub repository.
- Fine-tune the model on code data to improve its coding abilities.
- Build a web interface using Flask.
- Create a simple, user-friendly frontend for interacting with the AI.
What is Llama 3?
Llama 3 is Meta's latest large language model, trained on a vast amount of data—15 trillion tokens, to be precise. This powerful tool excels at understanding and generating human-like text, and it's specifically useful for applications like code generation, natural language understanding, and even creative writing.
As a cutting-edge LLM, Llama 3 sets new benchmarks in AI's ability to comprehend complex logic and generate contextually relevant responses. It is a versatile model, suitable for various AI-powered applications, especially those requiring high-level reasoning.
What is RAG (Retrieval-Augmented Generation)?
RAG, or Retrieval-Augmented Generation, is a technique that enhances the capabilities of Llama 3 by combining its text-generation abilities with real-time data retrieval. This allows Llama 3 to pull in relevant information from external sources, making its responses more informed and contextually appropriate. Whether you're generating code or writing essays, RAG can elevate the quality and accuracy of outputs.
If you're looking for a no-code way to implement RAG, platforms like Merlio AI offer simple tools for creating custom applications. This allows businesses to leverage advanced AI without needing extensive technical expertise.
Prerequisites
Before diving into the steps, ensure you have the following:
- Hardware Requirements: A computer with an NVIDIA GPU (8GB+ VRAM recommended).
- Software Requirements: Linux or Windows 10/11 operating system.
- Python: Make sure Python 3.7 or higher is installed.
- Basic Knowledge: Familiarity with Python and machine learning concepts.
Step 1: Install Llama 3
The first step is to set up Llama 3 on your local machine. It's open-source and available on GitHub, so you can easily clone the repository and get started.
Installation Commands:
bashCopy codegit clone https://github.com/facebookresearch/llama.git
cd llama
pip install -r requirements.txt
Once the repository is cloned, you'll need to download the pre-trained model weights from Meta's official website and place the .pth file in the llama/models directory.
Step 2: Fine-Tune Llama 3 on Code
To optimize Llama 3 for coding tasks, you'll need to fine-tune it on a dataset of code. One popular choice is the CodeSearchNet dataset, which contains a large set of code snippets and docstrings.
Download the Python Dataset:
bashCopy codewget https://s3.amazonaws.com/code-search-net/CodeSearchNet/v2/python.zip
unzip python.zip
Then, use the following script to fine-tune Llama 3 on the Python code dataset. This will help the model learn how to understand and generate Python code.
Fine-tuning Command:
bashCopy codepython finetune.py \
--base_model decapoda-research/llama-7b-hf \
--data_path python/train \
--output_dir python_model \
--num_train_epochs 3 \
--batch_size 128
This fine-tunes the model for three epochs on Python code and stores the resulting model in the python_model directory.
Step 3: Develop the Coding Assistant Interface
Now that we have a code-aware model, it’s time to create an interface for our AI coding assistant. We'll use Flask, a lightweight web framework, to build a simple web application.
Install Flask:
bashCopy codepip install flask
Create a Flask App:
Create a new file called app.py and add the following code to set up the backend API for your assistant:
pythonCopy codefrom flask import Flask, request, jsonify
from transformers import AutoModelForCausalLM, AutoTokenizer
app = Flask(__name__)
model_path = "python_model"
tokenizer = AutoTokenizer.from_pretrained(model_path)
model = AutoModelForCausalLM.from_pretrained(model_path)
@app.route('/complete', methods=['POST'])
def complete():
data = request.get_json()
code = data['code']
input_ids = tokenizer.encode(code, return_tensors='pt')
output = model.generate(input_ids, max_length=100, num_return_sequences=1)
generated_code = tokenizer.decode(output[0])
return jsonify({'generated_code': generated_code})
if __name__ == '__main__':
app.run()
This Flask app uses Llama 3 to generate code completions when a POST request is sent to the /complete endpoint.
Running the Flask App:
bashCopy codeflask run
Step 4: Create a Frontend UI
The next step is to build a simple user interface (UI) that will allow users to interact with the AI coding assistant. We’ll use a basic HTML file for this.
Create an index.html file:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>AI Coding Assistant</title>
</head>
<body>
<h1>AI Coding Assistant</h1>
<textarea id="code" rows="10" cols="50"></textarea>
<br>
<button onclick="complete()">Complete Code</button>
<p>Generated Code:</p>
<pre id="generated-code"></pre>
<script> function complete() { var code = document.getElementById("code").value; fetch('/complete', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({code: code}) }) .then(response => response.json()) .then(data => { document.getElementById("generated-code").textContent = data.generated_code; }); } </script>
</body>
</html>
This HTML page provides a simple interface where users can input code, send it to the backend, and view the generated code completion.
Deployment and Next Steps
Congratulations! You've now built a fully functional AI coding assistant using Llama 3. Here are some next steps you can take:
- Deploy your app: Consider deploying your app to platforms like AWS or Heroku so others can use it.
- Improve the frontend: Enhance the UI with features like syntax highlighting and multi-file support.
- Expand to other languages: Fine-tune Llama 3 for additional programming languages beyond Python.
- Experiment with alternatives: Try out different model architectures and hyperparameters to improve performance.
With the power of Llama 3, the possibilities for building AI-driven coding assistants are endless.
Frequently Asked Questions
Q: Can I use Llama 3 for languages other than Python?
Yes! While this guide uses Python for demonstration, you can fine-tune Llama 3 on any programming language by using a relevant dataset.
Q: How do I deploy my AI coding assistant?
You can deploy your app to cloud platforms such as AWS, Heroku, or even a local server. Just ensure your app is properly configured for cloud hosting.
Q: What other AI models can I use for coding assistants?
Besides Llama 3, you can explore models like GPT-4, Codex, or even fine-tuned versions of BERT for coding tasks.
Q: Do I need coding experience to use Llama 3?
Basic knowledge of Python and machine learning concepts is required, but advanced coding skills are not necessary if you're following this guide.
Explore more
U.S. Navy Bans DeepSeek AI Over National Security Concerns
The U.S. Navy has officially banned the use of Chinese AI startup DeepSeek due to rising national security concerns
Navigating the Ethical and Copyright Challenges of GitHub Copilot
Explore the ethical and copyright challenges surrounding GitHub Copilot. Learn about legal concerns, AI biases, and the ...
How Should AI Be Regulated? Key Insights and Global Developments
Explore the latest discussions on AI regulation, from California's AI bill to the EU AI Act. Learn about cybersecurity c...