December 24, 2024|6 min reading
How to Fix ModuleNotFoundError: No Module Named 'langchain_openai' Error
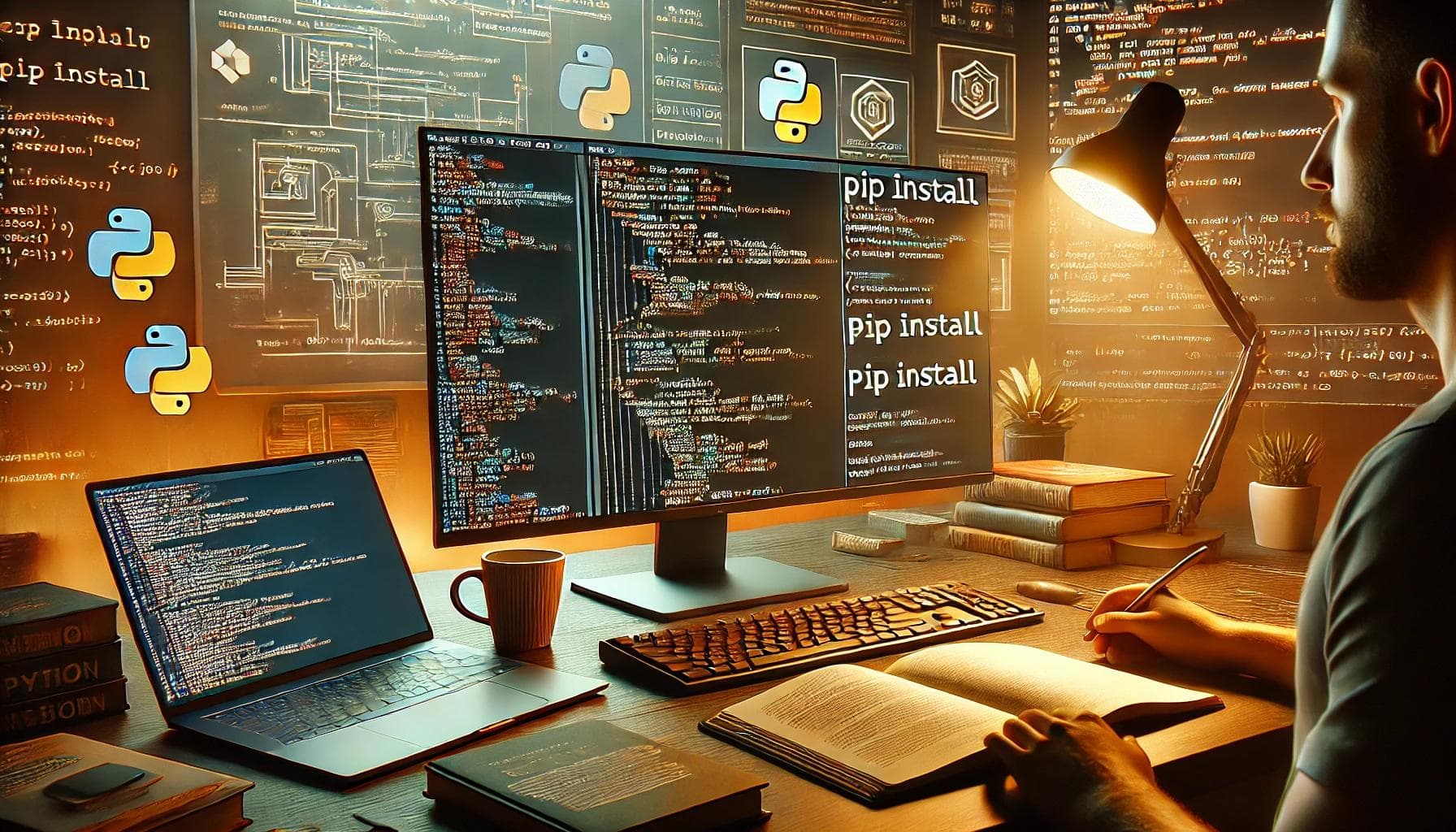
Don't Miss This Free AI!
Unlock hidden features and discover how to revolutionize your experience with AI.
Only for those who want to stay ahead.
Encountering the "ModuleNotFoundError: No module named 'langchain_openai'" error can be frustrating, especially if you’re trying to leverage LangChain for your AI projects. This guide explains the root cause of the issue, offers step-by-step solutions, and provides tips for avoiding similar errors in the future.
Understanding the LangChain OpenAI Error
This error typically arises from recent updates to the LangChain library. Starting with version 0.0.310, LangChain reorganized its structure by splitting functionalities into separate packages. This change improves maintainability but may require code and environment adjustments.
Steps to Resolve the LangChain OpenAI Error
Follow these steps to resolve the issue and get back on track.
Update LangChain and Related Packages
Ensure that you have the latest versions of LangChain and its dependencies by running the following commands:
pip install --upgrade langchain pip install --upgrade langchain-openai pip install --upgrade openai
These commands update LangChain, the LangChain OpenAI integration, and the OpenAI library to their most recent versions.
Install Additional Required Packages
Install any additional dependencies required for LangChain using the command below:
pip install langchain[all]
This installs LangChain with all optional dependencies, which helps avoid missing module issues.
Modify Your Import Statements
Update your import statements to match the new LangChain structure. Here’s how to do it:
Old Import:
from langchain.llms import OpenAI from langchain.chat_models import ChatOpenAI
New Import:
from langchain_openai import OpenAI from langchain_openai import ChatOpenAI
Check Your Python Environment
Make sure you’re using the correct Python environment where the updated packages are installed. If you’re using a virtual environment, activate it before running your script:
For Unix-based systems:
source venv/bin/activate
For Windows:
venv\Scripts\activate.bat
Verify Installation
Confirm the installation by running the following Python script:
import sys import langchain import langchain_openai import openai print(f"Python version: {sys.version}") print(f"LangChain version: {langchain.__version__}") print(f"LangChain OpenAI version: {langchain_openai.__version__}") print(f"OpenAI version: {openai.__version__}")
Ensure the printed versions match the latest releases.
Sample Code Using Updated LangChain OpenAI Integration
Here is a simple example demonstrating the updated LangChain OpenAI integration:
from langchain_openai import OpenAI from langchain.prompts import PromptTemplate from langchain.chains import LLMChain # Initialize the OpenAI language model llm = OpenAI(temperature=0.7) # Create a prompt template prompt = PromptTemplate( input_variables=["topic"], template="Write a short paragraph about {topic}." ) # Create an LLMChain chain = LLMChain(llm=llm, prompt=prompt) # Run the chain result = chain.run("artificial intelligence") print(result)
Troubleshooting Common Issues
If you’re still facing issues, here are some common problems and their solutions:
Issue 1: Conflicting Versions
Conflicting package versions may cause errors. Create a new virtual environment and install the packages afresh:
python -m venv new_langchain_env source new_langchain_env/bin/activate # Unix new_langchain_env\Scripts\activate.bat # Windows pip install langchain langchain-openai openai
Issue 2: Cached Imports
Python may cache imports, causing persistent issues. Restart your Python interpreter or IDE to resolve this.
Issue 3: System-wide vs. User Installation
Avoid mixing system-wide and user-specific installations. Use the --user flag if needed:
pip install --user langchain langchain-openai openai
Issue 4: Incompatible Dependencies
For projects with strict dependency requirements, create a requirements.txt file to manage versions:
Example requirements.txt:
langchain==0.0.311 langchain-openai==0.0.2 openai==0.27.8
Install from the file:
pip install -r requirements.txt
Best Practices for LangChain Development
Use Virtual Environments: Isolate dependencies to avoid conflicts.
Keep Dependencies Updated: Regularly update your packages to benefit from the latest features.
Version Control: Use a requirements.txt file or tools like Poetry for dependency management.
Refer to Documentation: Stay updated with LangChain’s official documentation.
Engage with the Community: Join forums or GitHub discussions for support.
Conclusion
The "ModuleNotFoundError: No module named 'langchain_openai'" error is a common but manageable issue. By following the steps and best practices in this guide, you can quickly resolve the error and continue building innovative AI applications with LangChain.
As LangChain evolves, staying updated and adapting your codebase will ensure seamless integration with cutting-edge AI tools. Keep exploring LangChain’s capabilities to unlock its full potential for your projects.
FAQ
Q1: Why am I still getting the error after updating packages? A: Ensure you’re using the correct Python environment and that the updated packages are installed in it. Restart your IDE to clear cached imports.
Q2: Can I avoid these errors in the future? A: Yes. Use virtual environments, manage dependencies carefully, and stay updated with official documentation.
Q3: What if I need an older version of LangChain? A: Use a requirements.txt file to specify the exact versions required and install using pip install -r requirements.txt.
Q4: Where can I find more information about LangChain updates? A: Check the official LangChain documentation and GitHub repository for the latest updates.
Q5: How can I learn more about LangChain’s features? A: Explore LangChain’s tutorials and community forums for detailed guides and best practices.
Explore more
U.S. Navy Bans DeepSeek AI Over National Security Concerns
The U.S. Navy has officially banned the use of Chinese AI startup DeepSeek due to rising national security concerns
Navigating the Ethical and Copyright Challenges of GitHub Copilot
Explore the ethical and copyright challenges surrounding GitHub Copilot. Learn about legal concerns, AI biases, and the ...
How Should AI Be Regulated? Key Insights and Global Developments
Explore the latest discussions on AI regulation, from California's AI bill to the EU AI Act. Learn about cybersecurity c...