December 25, 2024|7 min reading
How to Build a Local RAG App with Llama 3: A Complete Guide
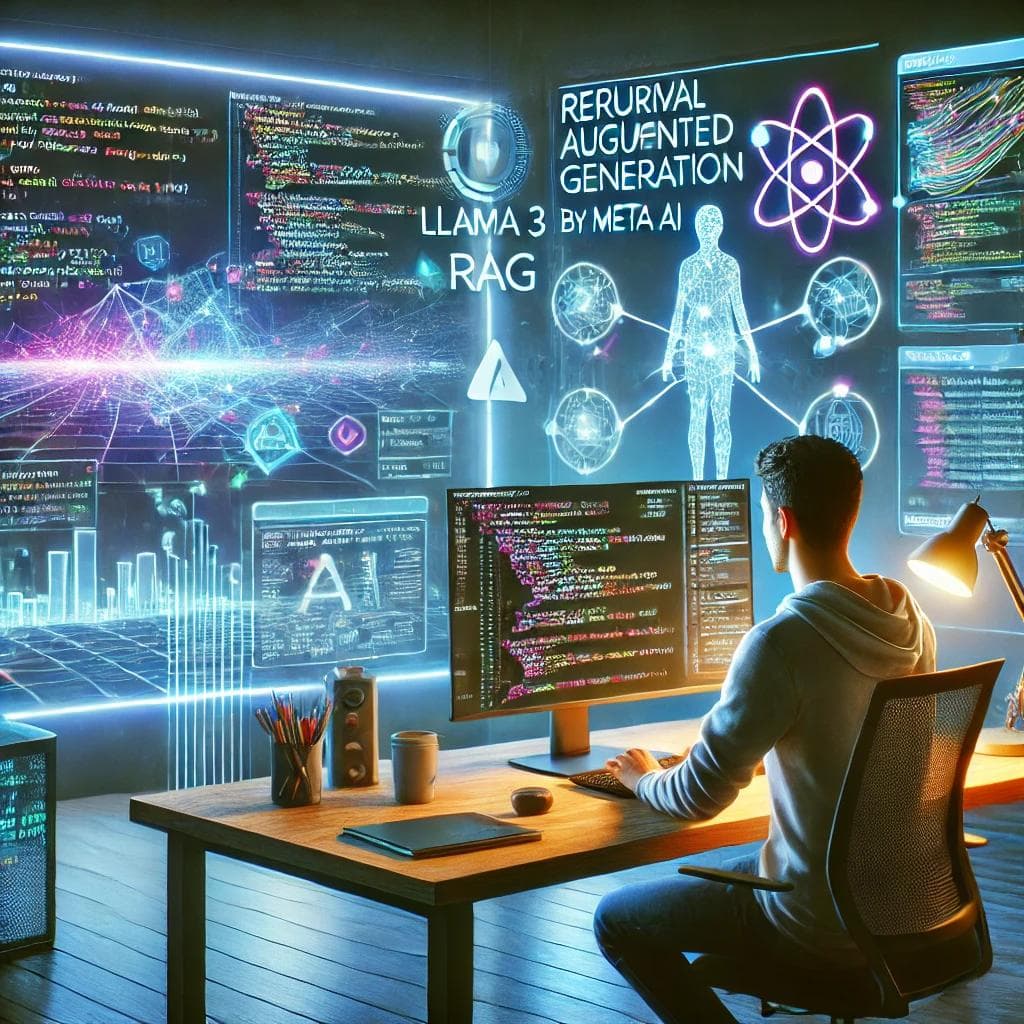
Don't Miss This Free AI!
Unlock hidden features and discover how to revolutionize your experience with AI.
Only for those who want to stay ahead.
In this comprehensive guide, we'll walk you through the process of building a Retrieval Augmented Generation (RAG) application using the state-of-the-art Llama 3 language model by Meta AI. This step-by-step tutorial will help you create a highly interactive app that can retrieve and generate responses based on the content of a webpage.
What is Llama 3?
Llama 3 is the latest language model developed by Meta AI. It is renowned for its ability to generate high-quality, context-aware text and engage in complex conversations. With its advanced natural language processing (NLP) capabilities, Llama 3 excels at understanding user queries and providing relevant, accurate responses.
What is RAG?
Retrieval Augmented Generation (RAG) is a hybrid AI technique that combines information retrieval with language generation. RAG systems retrieve data from a predefined knowledge base or documents and use that information to generate more accurate and contextually relevant responses. This makes it perfect for building smart applications that interact with users in a dynamic and informative manner.
Prerequisites for Building a Local Llama 3 RAG App
Before diving into the code, ensure you have the following installed on your system:
- Python 3.7 or higher
- Streamlit
- Ollama
- Langchain
- Langchain_community
You can easily install the necessary libraries by running the following command:
bashCopy codepip install streamlit ollama langchain langchain_community
Step-by-Step Guide to Build the App
Step 1: Set Up the Streamlit App
Start by creating a new Python file (app.py). This will be the foundation of your app. Here's the code to set up a basic Streamlit structure:
pythonCopy codeimport streamlit as st
import ollama
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain_community.document_loaders import WebBaseLoader
from langchain_community.vectorstores import Chroma
from langchain_community.embeddings import OllamaEmbeddings
st.title("Chat with Webpage 🌐")
st.caption("Interact with a webpage using Local Llama-3 and RAG.")
This sets up a basic title and caption, and a field where users can input the URL of the webpage they want to interact with.
Step 2: Load and Process the Webpage Data
Once the user enters the URL, we need to load and process the webpage data. Add the following code:
pythonCopy codeif webpage_url:
loader = WebBaseLoader(webpage_url)
docs = loader.load()
text_splitter = RecursiveCharacterTextSplitter(chunk_size=500, chunk_overlap=10)
splits = text_splitter.split_documents(docs)
This code loads the webpage data and splits it into manageable chunks to improve processing speed and accuracy.
Step 3: Create Ollama Embeddings and Vector Store
Next, create embeddings to enable the app to efficiently retrieve relevant information:
pythonCopy codeembeddings = OllamaEmbeddings(model="llama3")
vectorstore = Chroma.from_documents(documents=splits, embedding=embeddings)
Here, we're using Ollama embeddings and storing the vectorized documents in a Chroma vector store.
Step 4: Define the Ollama Llama-3 Model Function
Now we need to create a function that interacts with Llama-3 to generate responses based on the user's query:
pythonCopy codedef ollama_llm(question, context):
formatted_prompt = f"Question: {question}\n\nContext: {context}"
response = ollama.chat(model='llama3', messages=[{'role': 'user', 'content': formatted_prompt}])
return response['message']['content']
This function formats the user query along with relevant context and passes it to the Llama-3 model for a response.
Step 5: Set Up the RAG Chain
To retrieve relevant information from the vector store, set up the RAG chain:
pythonCopy coderetriever = vectorstore.as_retriever()
def combine_docs(docs):
return "\n\n".join(doc.page_content for doc in docs)
def rag_chain(question):
retrieved_docs = retriever.invoke(question)
formatted_context = combine_docs(retrieved_docs)
return ollama_llm(question, formatted_context)
This part allows the app to search through the stored data and generate answers based on the retrieved context.
Step 6: Implement the Chat Functionality
To enable users to interact with the webpage, implement a chat feature:
pythonCopy codeprompt = st.text_input("Ask a question about the webpage")
if prompt:
result = rag_chain(prompt)
st.write(result)
This lets users ask questions about the webpage, and the app will generate an answer using the RAG system.
Final Step: Time to Run the App!
Once everything is set up, run the app using the following command:
bashCopy codestreamlit run app.py
This will launch the app in your browser, and you can interact with the webpage through the Llama-3 model.
Conclusion
Congratulations! You've successfully built a RAG application using Llama 3. This local app allows users to engage in meaningful conversations with a webpage by retrieving relevant information and generating accurate responses. Now, feel free to enhance the app by adding more features or integrating additional functionalities.
FAQs
What is Llama 3?
Llama 3 is a state-of-the-art language model by Meta AI, designed to understand and generate human-like text based on context.
Do I need coding experience to run this app?
No, while coding is involved in the setup, you can follow the steps in this guide to set up the app on your local machine.
Can I deploy this app online?
Yes, you can deploy your Streamlit app on platforms like Heroku, AWS, or Google Cloud for online access.
What is the RAG technique?
Retrieval Augmented Generation (RAG) combines information retrieval with text generation to provide more accurate and contextually aware responses in AI systems.
Can I use other models besides Llama 3?
Yes, you can integrate other models like GPT or Claude into your app by adjusting the model settings in the code.
Explore more
Top 12 HeyGen AI Alternatives for 2025
Explore the 12 best HeyGen AI alternatives in 2025 for AI video generation. Find powerful platforms for realistic avatar...
Top 10 Free AI Voice Generators for Lifelike Audio
Easily convert text to speech, create realistic voiceovers for videos, podcasts, and more with these top tools
Claude MCP Server: Revolutionizing AI Interaction & Data Access
Discover how Claude MCP Server standardizes AI interaction with external data & tools. Learn its benefits for enterprise...